This week was all about adding Dawksin’s Pierce ability back in. There was quite a lot added to port projectiles to the new project so lets dive in.
The Base Projectile
Before I could even start porting Pierce, I needed to rebuild our base projectile.
“What is a base projectile?”
You
Basically, it’s a bunch of functionality that any projectile we create is going to share. This let’s us save time by not having to define how every single projectile:
- Moves
- Deals Damage
- Processes Collisions with Friendlies
- Processes Collisions with Enemies
- Gets destroyed on impact OR Impales on the enemies
- etc.
So let’s port over the basic functionality.
I started out in c++ land, to define what any projectile can do, no matter what type it is. This includes some really basic stuff like checking if it’s already hit something in the world so it doesn’t process it twice.

I tend to make most my objects very generic in case we need to change something in the future, so I added a way to fire events and define what information is passed in the blueprint itself.

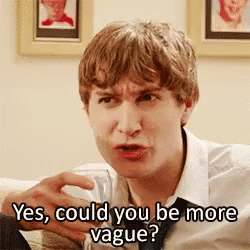
That should come in handy later…. Much later.
However, with that done, we can move on to the actual in-engine object.
From here I needed to make sure it can move, so a movement component was added with an initial speed. This gets us to our first step where the projectile can at least move.
The spawn doesn’t quite know where to place the projectile so by default it places it at the world origin (X: 0, Y: 0, Z: 0).
So to address that we’ll say the caster is where we want to spawn this projectile.

So we’ll wire it up again, and test.
So that’s great, let’s see how it work against enemies!
So from here we need to tweak a few things.
- Projectiles don’t detect enemies.
- Even if we do add collision detection, we need make sure we don’t hit the same target twice.
- It spawns right away, so we want to hide it until Dawksin’s hand is outstretched.
- If it can’t detect enemies, it can’t detect walls or the floor, so it can go through those.
- Needs to be Faster
So lets make those adjustments!
Tweaking the base projectile
We’ll add a collider, and check to see if we hit anything, if it doesn’t like us very much, we’ll try and deal some damage.

Next we’ll tackle the spawning problem. We’ll add some events to the animation when Dawksin’s hand is outstretched, and when he pulls back.
Then in the projectile we’ll add some listeners to respond to those events.
The main thing going on here is hiding it and stopping it’s movement.
(Also other performance considerations like stopping it from ticking. Which is a whole other post on its own, as performance stuff leaks into everything, and ticking is a big deal when you have a bunch of actors in the world, so you need to stop actors from ticking whenever they aren’t relevant, and back in my day we didn’t have-)

So anyway, lets see where we’re at.
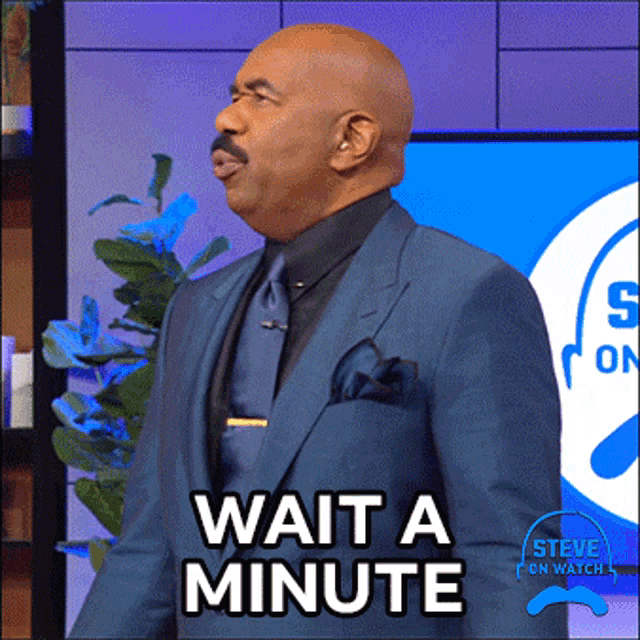
Why did we go through the trouble of making the projectile wait and then move? Sure it looks nice, but couldn’t we have just spawned it as soon as Dawksin’s hand reached out?
Well yes… but also no.
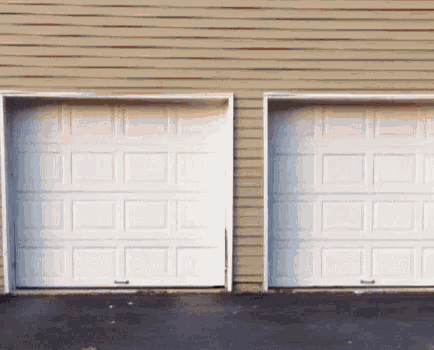
Because we’re multiplayer focused we need to think about what happens when any lag strikes. I was doing some reading a while back and this same scenario cropped up a long time ago. There was a great talk by Bungie on some methods they used for hiding lag in Halo. Lag is always going to be there, you just need to be smart about how you hide it.
So in Bungie’s case we can look at how they throw a grenade.
Picture this: You’re playing a round of Halo2 and you’re connected to your buddy. You go to throw a grenade.
A grenade toss animation plays, and a few frames later you see the grenade leave your hand.
You go to throw another grenade. BUT A LAG SPIKE HAPPENS!
You still play the grenade toss animation, and a second later, you see the grenade in the distance still landing where it should have.
This happens because the grenade spawns on your buddy’s machine, (The Host/Server) and you see the grenade when you hear back from their machine.
If you were allowed to spawn it on your machine, you could cheat, and spawn as many grenades as you wanted. So safer to spawn it on a trusted machine.
So we’re taking the same approach and spawning the Pierce projectile right away, that way you can have it in your world as soon as possible to hide some of the lag.
So now all that’s left to do is uh, you know, the rest of it.
Let’s add damage!
But Pierce has more heart than this. It’s very soul is missing.
THE RETURN PROJECTILE
Pierce returns to it’s caster, so we need to introduce a child class of our projectile.
The Homing Projectile. This class basically says, hey you can spawn me wherever, but tell me where to target and I’ll go from where I am to where I need to be!

Once we wire this up we get something that looks like this
So now it moves the return projectile to the initial projectile’s location, and then tells it to simulate. (Multiplayer: we also spawn this guy ahead of time, so technically both the initial projectile and the return projectile exist at the same time, they’re just deactivated until we need to use them)
We’ll add in collision detection and damage next.
VISUALS
Lets give pierce the look it deserves.
We’ll port over the mesh and particle systems, and wire them up where it makes sense. Since we don’t want the player to pay replication costs, we simulate anything locally wherever we can. In this case, since Dawksin is playing an animation on everyone’s machine we can stick the effects there.

The effect plays in a weird spot, so we’ll fix it up as soon as it spawns.

We’ll add another to the return projectile to complete the look

And finally we’ll wire it up to apply Dawksin’s Shrapnel stacks which we implemented in our last post.
So that’s fine and dandy, but it is a little hard to aim witho-
RETICLES
Normal humans can’t aim at the center of their screen all willy nilly.
Let’s give the player a reticle to help with aiming.
Here we import an icon for our reticle, and let it know it can change colors depending on who it’s over.
I’m not the best magician when it comes to making materials, so this one’s pretty simple.

Next we can make a widget to add on screen and give it this material. Complete with animations to change it’s color
Then we can tell it to either be center screen, or align with your lock target

The important bit is here were we compute it’s position, and check if we’re hovering over any characters

And I’m all out of time this week. I still need to make the hold version of Pierce, add camera shake (it really ties the room together), and add sounds.
But that should all be in by the next time I post.